If you look at the default CSS rules that define responsive layouts, it should be clear why the layouts don't work for printing:
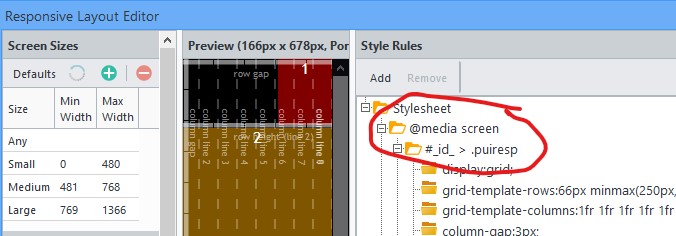
- Annotation 2020-03-04 093347.jpg (49.36 KiB) Viewed 670 times
. The rules are grouped under
CSS media selectors, meaning the rules only apply when the display medium is a screen. The Responsive Layout uses media selectors because there may be rules for different screen sizes.
In order to see things when printing, you could do one of these two:
1. you could manually edit the style rules in Visual Designer by selecting the layout and right-clicking on "style rules", and choosing "Open in Editor...".
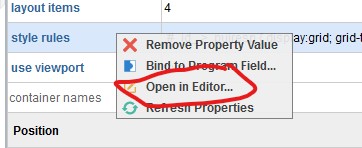
- Annotation 2020-03-04 094109.jpg (17.57 KiB) Viewed 670 times
Then remove the
and then remove the corresponding closing bracket. You could also copy the text and paste it into a better CSS text editor, edit it, and paste the changes back. This can be tricky if you have many rules. Also, this approach assumes that you have a default screen (that has no widths specified), and the default screen is how you want the layout to appear when printing--and that is probably not the case because screens tend to be wider than paper.
2. Add a global, default set of CSS rules for responsive layouts that would cause things to appear when printing anything in responsive layouts. The layout would not look the same as your screen, but it would appear. Something like this:
Code: Select all
@media print {
.puiresp {
width: 100%;
height: 100%;
display: grid;
}
.puiresp>div {
min-height: 50px;
min-width: 50px;
overflow: auto;
}
/* Overrule the absolute positioning of any widget's main tag if it's directly in container. */
.puiresp>div>[id] {
position: static !important;
}
/* Make the rows stack on top of each other. (Supports up to 15 rows.) */
.puiresp>div:nth-of-type(1) { grid-row: 1; }
.puiresp>div:nth-of-type(2) { grid-row: 2; }
.puiresp>div:nth-of-type(3) { grid-row: 3; }
.puiresp>div:nth-of-type(4) { grid-row: 4; }
.puiresp>div:nth-of-type(5) { grid-row: 5; }
.puiresp>div:nth-of-type(6) { grid-row: 6; }
.puiresp>div:nth-of-type(7) { grid-row: 7; }
.puiresp>div:nth-of-type(8) { grid-row: 8; }
.puiresp>div:nth-of-type(9) { grid-row: 9; }
.puiresp>div:nth-of-type(10) { grid-row: 10; }
.puiresp>div:nth-of-type(11) { grid-row: 11; }
.puiresp>div:nth-of-type(12) { grid-row: 12; }
.puiresp>div:nth-of-type(13) { grid-row: 13; }
.puiresp>div:nth-of-type(14) { grid-row: 14; }
.puiresp>div:nth-of-type(15) { grid-row: 15; }
}
The advantage to this is that it requires no changes to individual display files, and each block will be visible. The disadvantage is that things will not appear how you designed them. Put them in a .css file under /www/<yourinstancename>/htdocs/profoundui/userdata/custom/css/.
I think those @media print rules need to be added to the default Profound UI css stylesheet. I'll look into it. You can also ask support at profoundlogic.com to add a feature for designing for print media using the responsive layout editor.
Edited: changed CSS "display: block;" to "display: grid;"